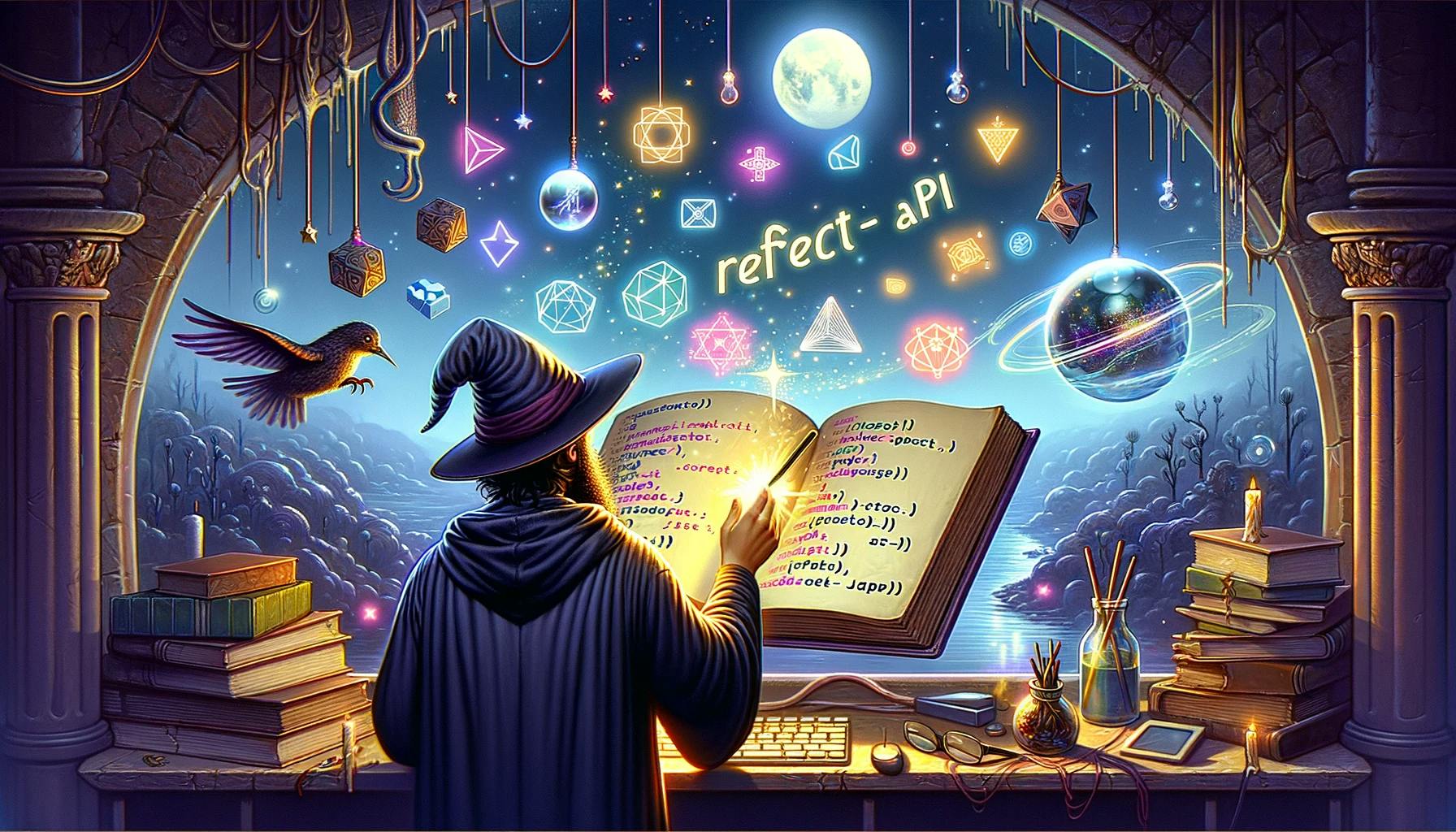
Unleashing the Power of JavaScript's Reflect API with a Pokémon Twist
Hey fellow coders and Pokémon trainers! Have you ever wished you had a Pokédex for JavaScript objects, letting you inspect and interact with them in ways you never thought possible? Well, your wish is granted with the magical world of the Reflect API. This underappreciated gem in the JavaScript language is like having a psychic Pokémon by your side, giving you extraordinary powers to manipulate objects in real-time. So, let's embark on this adventure together and explore the wonders of the Reflect API, Pokémon style!
What is the Reflect API?
Introduced in ECMAScript 2015 (ES6), the Reflect API is a built-in object with static methods for interceptable JavaScript operations, similar to those we could proxy. Think of it as a toolbox that allows you to perform object operations that you usually do (like getting/setting properties or calling a function) but with a whole new level of control and flexibility. It's like having a Master Ball; it just gives you that extra edge.
Why Reflect? Why Now?
You might wonder, "Why should I bother with Reflect when I've been doing just fine without it?" Well, just like in the world of Pokémon, where understanding the strengths and weaknesses of different types can be the key to becoming a Pokémon Master, understanding and using the Reflect API can help you write cleaner, more flexible, and maintainable code. It's about upgrading your toolkit and embracing the full potential of JavaScript.
A Reflective Journey with Our Pokémon Friends
Let's dive into some examples of how the Reflect API can be as helpful as having a Pikachu by your side during a stormy battle.
Reflect.get
: Peeking into an Object
Imagine you're on a quest to find a rare Pokémon. You've heard rumors about its last known location, but you need to confirm. In JavaScript, this is like trying to access a property of an object.
const pikachu = {
name: 'Pikachu',
type: 'Electric',
level: 42,
};
const propName = 'type';
console.log(Reflect.get(pikachu, propName)); // Outputs: Electric
Using Reflect.get, we can dynamically access the type property of our Pikachu. It's like using a Pokédex to find out the type of Pokémon you've just encountered.
Reflect.set
: Teaching Your Pokémon New Moves
Now, suppose you want to teach your Pikachu a new move. Normally, you'd directly assign a new value to a property, but with Reflect, you can do this more thoughtfully.
const move = 'Thunderbolt';
Reflect.set(pikachu, 'move', move);
console.log(pikachu.move); // Outputs: Thunderbolt
This is akin to teaching Pikachu the Thunderbolt move. With Reflect.set, we've added a new move to Pikachu's repertoire, dynamically and flexibly.
Reflect.deleteProperty
: Letting Go of Unwanted Moves
Sometimes, a Pokémon might need to forget a move to make room for a new one. Similarly, you might need to delete a property from an object.
Reflect.deleteProperty(pikachu, 'level');
console.log(pikachu); // Outputs: { name: 'Pikachu', type: 'Electric', move: 'Thunderbolt' }
It's as if we visited the Move Deleter to forget an old move. Reflect.deleteProperty lets us modify our object on the fly, no heart scales required!
Reflect.has
: Checking for Hidden Abilities
Curious if your Pokémon has a hidden ability? In JavaScript, checking if an object has a certain property is a breeze with Reflect.
console.log(Reflect.has(pikachu, 'type')); // Outputs: true
This is like asking the Pokédex, "Hey, does Pikachu have a type?" And of course, the answer is a resounding yes!
The Adventure Continues
The examples above are just the beginning of what's possible with the Reflect API. Like exploring every nook and cranny of the Pokémon world, there's so much more to discover with Reflect. Methods like:
Reflect.construct
for creating new instances (imagine hatching a new Pokémon).Reflect.apply
for calling functions (like commanding your Pokémon in battle).Reflect.defineProperty
(equipping your Pokémon with a new item) open up a universe of possibilities.
Conclusion: Why Reflect Deserves a Spot in Your Team?
Just as every Pokémon has its place in your journey to becoming a Pokémon Master, the Reflect API has its place in the world of JavaScript. It offers a level of interaction with objects that's not only powerful but also incredibly flexible, making your code more adaptable and robust. Whether you're a seasoned developer or just starting out, giving the Reflect API a try might just reveal hidden potentials in your code, much like discovering the hidden potential in your Pokémon team.