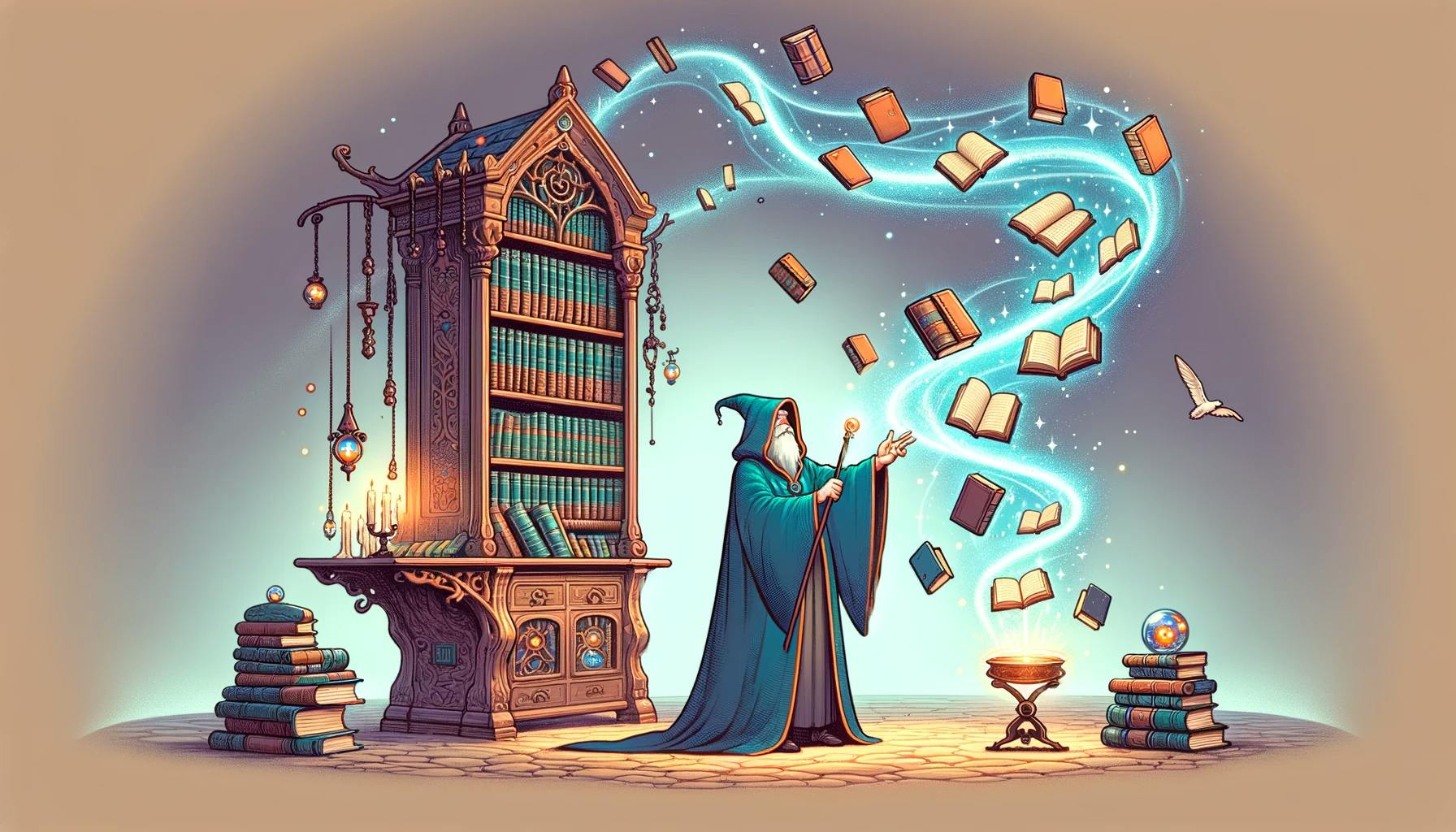
Mastering JavaScript Proxies for Smarter Web Development
Welcome, web wizards and coding conjurers! Today, we pull back the curtain on one of JavaScript's most powerful and mysterious features: the Proxy object. With this arcane artifact, you can create a powerful abstraction layer over your objects, opening a realm of meta-programming that allows you to intercept and redefine fundamental language operations. No need for enchanted scrolls or ancient incantations; JavaScript Proxies are all you need to add a touch of sorcery to your code!
A Gateway to JavaScript Meta-programming: Understanding Proxies
In the realm of JavaScript, a Proxy is a special object that wraps another object or function and intercepts the interactions with that target. It's like having an intelligent agent that stands between you and the object, controlling access and behavior. You define this behavior through handlers, which are like spells that can manipulate property access, assignment, enumeration, and function invocation.
Here's a spellbook to conjure a simple proxy:
const wizard = {
name: 'Merlin',
age: 'immortal'
};
const agent = new Proxy(wizard, {
get(target, property) {
if (property in target) {
return target[property];
}
return 'Property not found in the wizard\'s memory.';
}
});
console.log(agent.name); // Outputs: Merlin
console.log(agent.age); // Outputs: immortal
console.log(agent.spell); // Outputs: Property not found in the wizard's memory.
The Power of Interception: Practical Uses of Proxies
Proxies are not just for show; they hold practical powers that can enhance your JavaScript projects. Let's conjure up some real-world examples.
Validation
Proxies can ensure that objects only accept valid data. Like a guardian golem, a Proxy can protect an object from invalid manipulations.
function createGuardian(target, validator) {
return new Proxy(target, {
set(obj, prop, value) {
if (validator(prop, value)) {
obj[prop] = value;
return true;
}
throw new Error(`The crystal ball says that "${value}" is not a valid value for "${prop}".`);
}
});
}
const wizardStats = createGuardian({}, (prop, value) => typeof value === 'number');
wizardStats.strength = 10;
console.log(wizardStats.strength); // Outputs: 10
// This will throw an error because 'mighty' is not a number
wizardStats.strength = 'mighty';
Access Control
Control read/write access to certain properties with a Proxy, like a secretive guild that only allows members access to its hidden knowledge.
const secretDiary = {
_secret: "I'm afraid of water."
};
const diaryProxy = new Proxy(secretDiary, {
get(target, property, receiver) {
if (property.startsWith('_')) {
throw new Error('Access denied to secret entries.');
}
return Reflect.get(target, property, receiver);
},
set(target, property, value, receiver) {
if (property.startsWith('_')) {
throw new Error('It’s forbidden to write secret entries.');
}
return Reflect.set(target, property, value, receiver);
}
});
console.log(diaryProxy._secret); // Throws Error
Profiling and Debugging
Wrap functions with a Proxy to keep track of how often and with what arguments they're called, akin to an arcane scry that monitors spell usage.
function profileFunction(func) {
return new Proxy(func, {
apply(target, thisArg, argumentsList) {
console.log(`Summoned with arguments: ${argumentsList}`);
console.time('Execution time');
const result = Reflect.apply(target, thisArg, argumentsList);
console.timeEnd('Execution time');
return result;
}
});
}
const sum = profileFunction((...nums) => nums.reduce((a, b) => a + b, 0));
sum(1, 2, 3); // Console will show the arguments and the execution time
Proxies and Reflection: A Match Made in the Heavens
JavaScript's Reflect object works hand-in-hand with Proxies, providing default actions for the intercepted operations. Think of Reflect methods as the natural laws of the JavaScript universe, offering a safe way to perform the original object behavior.
Conclusion: The Art of Proxy Magic
JavaScript Proxies open the door to a world of possibilities, from the creation of smart and dynamic data models to clever tricks that can simplify and enhance your code. Like any advanced feature, they should be used with care — an irresponsible spellcaster can easily create confusing abstractions or performance pitfalls.
Now, go forth and harness the power of JavaScript Proxies to bend the digital cosmos to your will! May your data always be validated, your access controlled, and your functions expertly profile